43 python tkinter change label text
python 3.x - How to change the text color using tkinter.Label - Stack ... import tkinter as tk root = tk.tk () # bg is to change background, fg is to change foreground (technically the text color) label = tk.label (root, text="what's my favorite video?", bg='#fff', fg='#f00', pady=10, padx=10, font=10) # you can use use color names instead of color codes. label.pack () click_here = tk.button (root, text="click here … python - Changing Tkinter Label Text Dynamically using Label.configure ... After you change the text to "Process Started", use label.update (). That will update the text before sleep ing for 5 seconds. Tkinter does everything in its mainloop, including redrawing the text on the label. In your callback, it's not able to draw it because your callback hasn't returned yet.
Python Tkinter - Label - GeeksforGeeks Label Widget. Tkinter Label is a widget that is used to implement display boxes where you can place text or images. The text displayed by this widget can be changed by the developer at any time you want. It is also used to perform tasks such as to underline the part of the text and span the text across multiple lines.
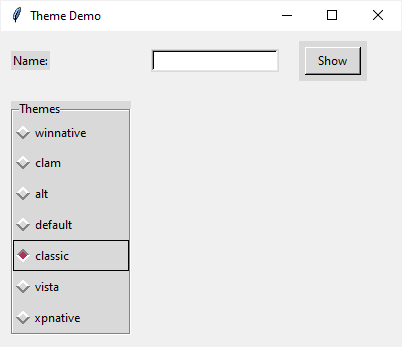
Python tkinter change label text
How to align text to the left in Tkinter Label? - tutorialspoint.com #Import the required library from tkinter import* #Create an instance of tkinter frame win= Tk() #Set the geometry win.geometry("750x250") #Create a Label Widget Label(win, text= "New Line Text", font= ('Helvetica 15 underline'), background="gray74").pack(pady=20, side= TOP, anchor="w") win.mainloop() Output How to change the Tkinter label text | Code Underscored Apr 06, 2022 · import tkinter as tk class Test(): def __init__(self): self.root = tk.Tk() self.text = tk.StringVar() self.text.set("Test") self.label = tk.Label(self.root, textvariable=self.text) self.button = tk.Button(self.root, text="Click to change text below", command=self.changeText) self.button.pack() self.label.pack() self.root.mainloop() def changeText(self): self.text.set("Text updated") app=Test() self.text = tk.StringVar() self.text.set("Code Test") How to change the Tkinter label text? - GeeksforGeeks Aug 17, 2022 · Click here For knowing more about the Tkinter label widget. Now, let’ see how To change the text of the label: Method 1: Using Label.config() method. Syntax: Label.config(text) Parameter: text– The text to display in the label. This method is used for performing an overwriting over label widget. Example:
Python tkinter change label text. How to update the image of a Tkinter Label widget? - tutorialspoint.com A Label widget takes text and images in the constructor that sets the label with the position in the top-left corner of the window. However, to change or update the image associated with the Label, we can use a callable method where we provide the information of other images. python - Changing the text on a label - Stack Overflow You can also define a textvariable when creating the Label, and change the textvariable to update the text in the label. Here's an example: labelText = StringVar () depositLabel = Label (self, textvariable=labelText) depositLabel.grid () def updateDepositLabel (txt) # you may have to use *args in some cases labelText.set (txt) Tkinter Label - Python Tutorial First, import Label class from the tkinter.ttk module. Second, create the root window and set its properties including size, resizeable, and title. Third, create a new instance of the Label widget, set its container to the root window, and assign a literal string to its text property. Setting a specific font for the Label Change the Tkinter Label Text | Delft Stack Nov 25, 2019 · Another solution to change the Tkinter label text is to change the text property of the label. import tkinter as tk class Test(): def __init__(self): self.root = tk.Tk() self.label = tk.Label(self.root, text="Text") self.button = tk.Button(self.root, text="Click to change text below", command=self.changeText) self.button.pack() self.label.pack() self.root.mainloop() def changeText(self): self.label['text'] = "Text updated" app=Test()
How to Change Label Text on Button Click in Tkinter Another way to change the text of the Tkinter label is to change the 'text' property of the label. import tkinter as tk def changeText(): label['text'] = "Welcome to StackHowTo!" gui = tk.Tk() gui.geometry('300x100') label = tk.Label(gui, text="Hello World!") label.pack(pady=20) button = tk.Button(gui, text="Change the text", command=changeText) Update Label Text in Python TkInter - Stack Overflow May 12, 2017 · from Tkinter import Tk, Checkbutton, Label from Tkinter import StringVar, IntVar root = Tk() text = StringVar() text.set('old') status = IntVar() def change(): if status.get() == 1: # if clicked text.set('new') else: text.set('old') cb = Checkbutton(root, variable=status, command=change) lb = Label(root, textvariable=text) cb.pack() lb.pack() root.mainloop() Change the Tkinter Label Text - zditect.com self.label = tk.Label (self.root, textvariable=self.text) It associates the StringVar variable self.text to the label widget self.label by setting textvariable to be self.text. The Tk toolkit begins to track the changes of self.text and will update the text self.label if self.text is modified. The above code creates a Tkinter dynamic label. How to Change the Tkinter Label Font Size? - GeeksforGeeks Label ( self.master, text="I have a font-size of 25", # Changing font-size using custom style style="My.TLabel").pack () if __name__ == "__main__": # Instantiating top level root = Tk () # Setting the title of the window root.title ("Change font-size of Label") # Setting the geometry i.e Dimensions root.geometry ("400x250") # Calling our App
Setting the position of TKinter labels - GeeksforGeeks Label: Tkinter Label is a widget that is used to implement display boxes where you can place text or images. The text displayed by this widget can be changed by the developer at any time you want. It is also used to perform tasks such as to underline the part of the text and span the text across multiple lines. Python tkinter Basic: Create a label and change the label font style ... Write a Python GUI program to create a label and change the label font style (font name, bold, size) using tkinter module. Sample Solution: Python Code: import tkinter as tk parent = tk.Tk() parent.title("-Welcome to Python tkinter Basic exercises-") my_label = tk.Label(parent, text="Hello", font=("Arial Bold", 70)) my_label.grid(column=0, row ... How to update a Python/tkinter label widget? - tutorialspoint.com Output. Running the above code will display a window that contains a label with an image. The Label image will get updated when we click on the "update" button. Now, click the "Update" button to update the label widget and its object. Rename the label in tkinter - Python - Tutorialink Rename the label in tkinter. I work with tkinter. I want to change the name of the labels. By entering the characters in the field and hitting the button, the labels are renamed one by one. That is, the first time I enter the character "Hello", then that character is inserted in the label; It is then removed from the field.
How to Set Border of Tkinter Label Widget? - GeeksforGeeks Set a label widget with required attributes for border. Place this widget on the window created. Syntax: Label ( master, option, …. ) Parameters: Master: This represents the parent window. Option: There are so many options for labels like bg, fg, font, bd, etc. Now to set the border of the label we need to add two options to the label property:
Change label (text) color in tkinter | Code2care Output: tkinter - Change label text color. ⛏️ You can use color names such as - black, white, green, yellow, orange, etc. ⛏️ You can also use hex color code just like you may use with HTML or CSS: Example: #eeeeee, #202020. Tkinter - add x and y padding to label text. Validate email address in Python using regular expression (regex)
python 3.x - Tkinter - Changing label text via another function - Stack ... def ChangeLabelText (m): m.config (text = 'You pressed the button!') And withing main () you will need to pass MyLabel as an argument to ChangeLabelText ().
How to change Tkinter label text on button press? - tutorialspoint.com # import the required libraries from tkinter import * # create an instance of tkinter frame or window win = tk() # set the size of the tkinter window win.geometry("700x350") # define a function update the label text def on_click(): label["text"] = "python" b["state"] = "disabled" # create a label widget label = label(win, text="click the button …
Changing Tkinter Label Text Dynamically using Label.configure() # import the required library from tkinter import * # create an instance of tkinter frame or widget win = tk () win. geometry ("700x350") def update_text(): # configuring the text in label widget label. configure ( text ="this is updated label text") # create a label widget label = label ( win, text ="this is new label text", font =('helvetica 14 …
Python Set Label Text on Button Click tkinter GUI Program Create a label with empty text. Place label in the main window at x,y location. Define and set a txt variable to "Easy Code Book" string literal. Define btn1_click () function to handle the button click event. Set the text of label to txt variable. Create a button and bind it to btn1_click event handler by setting command option.
How to dynamically add/remove/update labels in a Tkinter window? To dynamically update the Label widget, we can use either config (**options) or an inline configuration method such as for updating the text, we can use Label ["text"]=text; for removing the label widget, we can use pack_forget () method. Example
Update Tkinter Label from variable - tutorialspoint.com To display the text and images in an application window, we generally use the Tkinter Label widget. In this example, we will update the Label information by defining a variable. Whenever the information stored in the variable changes, it will update the Label as well. We can change the Label information while defining the textvariable property ...
Tkinter Change Label Text - Linux Hint text = "Tkinter Change Label Text") label1. pack() button1. pack() window1. mainloop() You can see the label and the button in the following output screen. When we click on the button, the label is successfully updated, as you can see. Example 3:
How to change the Tkinter label text? - GeeksforGeeks Aug 17, 2022 · Click here For knowing more about the Tkinter label widget. Now, let’ see how To change the text of the label: Method 1: Using Label.config() method. Syntax: Label.config(text) Parameter: text– The text to display in the label. This method is used for performing an overwriting over label widget. Example:
How to change the Tkinter label text | Code Underscored Apr 06, 2022 · import tkinter as tk class Test(): def __init__(self): self.root = tk.Tk() self.text = tk.StringVar() self.text.set("Test") self.label = tk.Label(self.root, textvariable=self.text) self.button = tk.Button(self.root, text="Click to change text below", command=self.changeText) self.button.pack() self.label.pack() self.root.mainloop() def changeText(self): self.text.set("Text updated") app=Test() self.text = tk.StringVar() self.text.set("Code Test")
How to align text to the left in Tkinter Label? - tutorialspoint.com #Import the required library from tkinter import* #Create an instance of tkinter frame win= Tk() #Set the geometry win.geometry("750x250") #Create a Label Widget Label(win, text= "New Line Text", font= ('Helvetica 15 underline'), background="gray74").pack(pady=20, side= TOP, anchor="w") win.mainloop() Output
Post a Comment for "43 python tkinter change label text"